About redis
redis is data structure server It’s opensource, Key-value database ,networked, in-memory.
Data types is maps keys to types of values.
Redis supports strings and abstract data types.
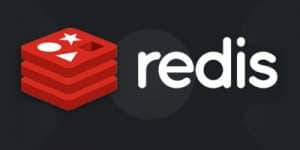
Install redis on ubuntu 14.04
- install redis server
sudo apt-get install redis-server - you can check progress and check port
ps -aux|grep redis
netstat -nlt|grep 6379 - check status
/etc/init.d/redis-server status
or
service redis-server status
CLI
documentation: http://redis.io/commands
- run cli
redis-cli - help tip
help
Type: “help @” to get a list of commands in
“help” for help on
“help ” to get a list of possible help topics
“quit” to exit - show all key list
keys *
- add key value
set key1 “hi cake!”
OK - show key value
get key1
“hi cake!” - add key number
set key2 1
OK - Increment number
INCR key2
(integer) 2 - add list data
LPUSH key3 jeff
LPUSH key3 cake
LPUSH key3 ken - show list data
LRANGE key3 0 3
- add hash tables
HSET key4 name “golden cake”
HSET key4 email “jeff@golden-cake.com“ - get hash tables by key
HGET key4 name
“golden cake” - get all hash tables
HGETALL key4
1) “name”
2) “golden cake”
3) “email”
4) “jeff@golden-cake.com“ - create hash tables by more key and value
HMSET key5 username jeff password 123456 mail jeff@golden-cake.com
- show name and mail
HMGET key5 username mail
- show all
HGETALL key5
1) “username”
2) “jeff”
3) “password”
4) “123456”
5) “mail”
6) “jeff@golden-cake.com“ - show all key
keys *
1) “key2”
2) “key5”
3) “key4”
4) “key1”
5) “key3” - remove key1 and key5
del key1 key5
- remove all key
FLUSHALL
set password
- vim /etc/redis/redis.conf
- enable requirepass [yourpassword]
- service redis-server restart
- >redis-cli -a [yourpassword]
If you want to remotely access the database
- vim /etc/redis/redis.conf
- disable bind 127.0.0.1
- service redis-server restart
- redis-cli -a [yourpassword] -h xxx.xxx.xxx.xxx
How to working on php7-fpm
- download phpredis source code
git clone https://github.com/phpredis/phpredis.git
cd phpredis - Switch to php7 branch
git checkout php7
- Compile
phpize
./configure
make && make install
cd ..
rm -rf phpredis - create php7 config :vim /etc/php/7.0/fpm/conf.d/30-redis.ini
extension=redis.so
- restart php7-fpm and check phpinfo
service php7.0-fpm restart
Test connection Redis
documentation: https://github.com/phpredis/phpredis#classes-and-methods
- connect
”’
<?php
\(redis = new Redis();
\)redis->connect(‘127.0.0.1′, 6379);
echo “Connection to server sucessfully”;
echo “Server is running: ” . $redis->ping();
”’
Working With Node.js and Redis
documentation: http://redis.js.org/
- install node_redis
npm install redis
- connect
”’
var redis = require(“redis”),
client = redis.createClient();//port and ip
//client = redis.createClient(‘6379’, ‘127.0.0.1’);//auth
// client.auth(“passowrd”);
//error
client.on(“error”, function (err) {
console.log(“Error ” + err);
});
”’